Building a Universal Social Media Embed Component in React Jodit
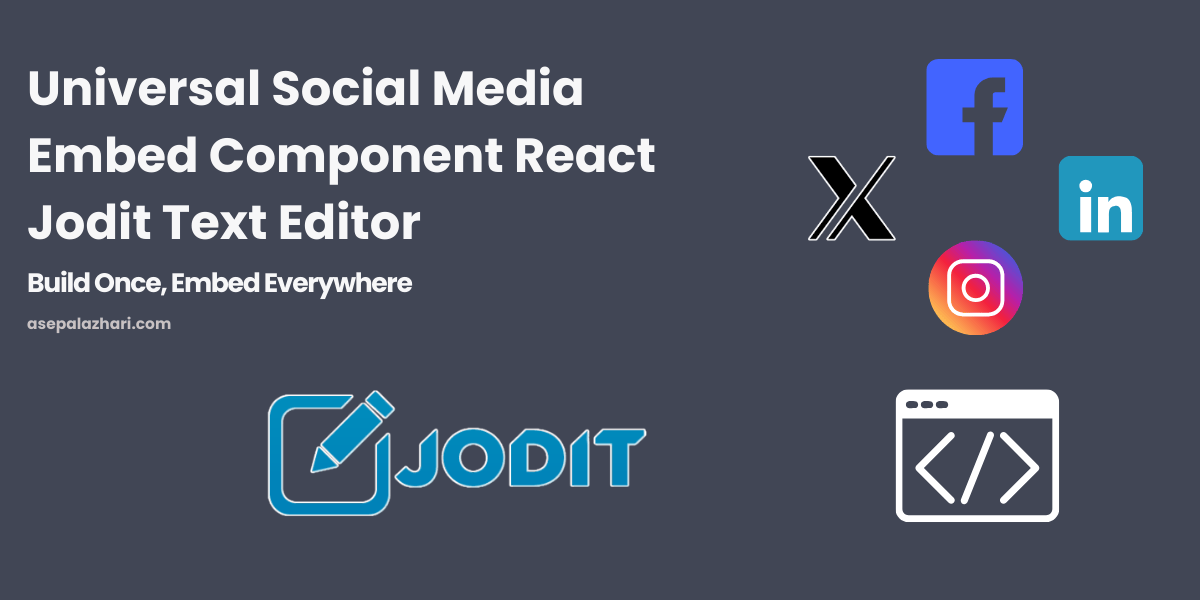
In today's interconnected digital landscape, integrating social media content into web applications has become a crucial requirement for many projects. Whether you're building a content management system, a blog platform, or a news website, the ability to seamlessly embed social media posts can significantly enhance user engagement and content richness.
The Challenge of Social Media Embedding
When I first faced the task of implementing social media embeds across multiple platforms, I quickly realized that each platform had its own unique implementation requirements, loading patterns, and initialization needs. What started as a simple feature request soon became a complex juggling act of managing different script loading strategies, handling platform-specific callbacks, and maintaining a consistent user experience.
This is where the idea for a universal social media embed component was born – a single, reusable solution that could handle multiple platforms while providing a smooth, user-friendly experience.
Also Read: Best Text Editors for React: CKEditor vs TinyMCE vs Jodit
Why Create a Universal Embed Component?
Before diving into the implementation, let's understand the key benefits of creating a universal embed component:
- Consistency: A unified interface for embedding content from any supported platform
- Maintainability: Centralized error handling and loading states
- Type Safety: Full TypeScript support for better development experience
- User Experience: Smooth loading states and error feedback
- Extensibility: Easy to add support for new platforms
Additionally, we will explore how to integrate this custom embed component as a plugin within the Jodit Editor for React, enhancing content creation workflows.
Key Features
- Platform Agnostic: Seamlessly embeds content from multiple social media platforms.
- TypeScript Support: Ensures robust type checking.
- Loading States: Enhances user experience by indicating content loading status.
- Error Handling: Manages API errors gracefully.
- Jodit Editor Integration: Enables embedding directly within a rich text editor.
Prerequisites
- Basic understanding of React and TypeScript.
- Familiarity with social media API integration.
- Experience with Jodit Editor is helpful but not required.
Also Read: Add Header in Image Next.js: A Comprehensive Guide
Project Setup
Step 1: Create a New React Project
npx create-react-app social-media-embed --template typescript
cd social-media-embed
Step 2: Install Required Packages
You'll need to install Axios for API requests and Jodit Editor:
npm install axios jodit-react
Step 3: Component Structure
Create a SocialMediaEmbed.tsx
file in the src/components
directory:
import React, { useState, useEffect } from "react";
import axios from "axios";
interface EmbedProps {
url: string;
}
const SocialMediaEmbed: React.FC<EmbedProps> = ({ url }) => {
const [content, setContent] = useState<string | null>(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState<string | null>(null);
useEffect(() => {
const fetchContent = async () => {
try {
const response = await axios.get(
`https://api.embed-service.com?url=${encodeURIComponent(
url
)}`
);
setContent(response.data.html);
} catch (err) {
setError("Failed to load content.");
} finally {
setLoading(false);
}
};
fetchContent();
}, [url]);
if (loading) return <p>Loading...</p>;
if (error) return <p>{error}</p>;
return <div dangerouslySetInnerHTML={{ __html: content || "" }} />;
};
export default SocialMediaEmbed;
Step 4: Integrating with Jodit Editor
To use this component as a custom plugin in Jodit Editor, create a custom button that inserts the embed component.
Jodit Plugin Example
import React from "react";
import JoditEditor from "jodit-react";
import SocialMediaEmbed from "./SocialMediaEmbed";
const EditorWithEmbedPlugin: React.FC = () => {
const editorConfig = {
toolbarButton: ["embed"],
buttons: [
{
name: "embed",
iconURL:
"https://icon-library.com/images/embed-icon/embed-icon-16.jpg",
exec: (editor: any) => {
editor.selection.insertHTML(
'<div id="embed-placeholder"></div>'
);
},
},
],
};
return (
<JoditEditor
config={editorConfig}
onChange={(newContent) => console.log(newContent)}
/>
);
};
export default EditorWithEmbedPlugin;
Conclusion
This component provides a simple yet powerful solution for embedding social media content in your React applications and integrating it as a custom plugin for Jodit Editor. With support for TypeScript, loading states, and error handling, it ensures a seamless user experience.
Remember that social media platforms frequently update their embedding mechanisms, so it's important to keep your implementation up to date with the latest platform changes and requirements.