Add Header in Image Next.js: A Comprehensive Guide
Securely load images in Next.js by adding custom headers and using the Secure Image component. Get performance tips & practical examples!
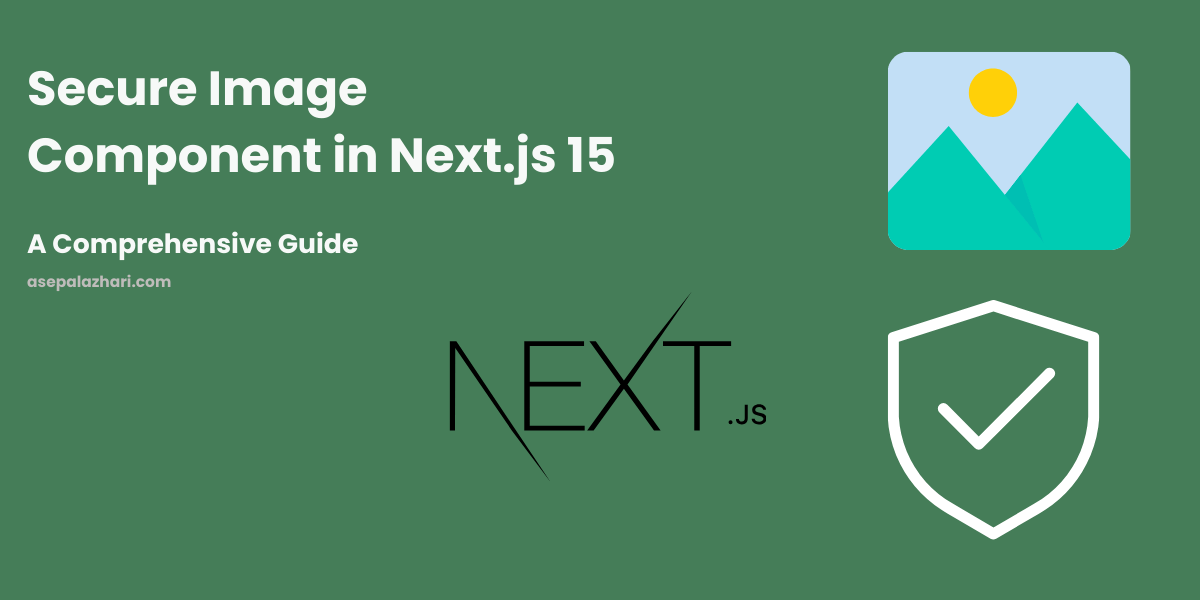
In modern web applications, handling authenticated or token-protected images is a common challenge. Whether you’re working with private content management systems, secure file repositories, or restricted asset libraries, you’ll often encounter scenarios where images require special authentication to access.
The Challenge of Secure Image Loading
Traditional image-loading methods often fall short when dealing with images requiring custom headers or authentication tokens. While Next.js provides a powerful built-in Image
component for optimized image handling, it doesn’t natively support scenarios involving complex authentication requirements.
Our Solution: A Custom Secure Image Component
To address this, we’ll create a robust solution for secure image loading in Next.js. Here’s how:
import Image from "next/image";
import { useState, useEffect } from "react";
const SecureImage = ({ src, token, alt, width, height }) => {
const [secureImageSrc, setSecureImageSrc] = useState(null);
useEffect(() => {
const fetchSecureImage = async () => {
try {
const response = await fetch(src, {
headers: {
"X-Secure-File-Token": token,
},
});
if (!response.ok) {
throw new Error("Failed to fetch secure image");
}
const blob = await response.blob();
const imageUrl = URL.createObjectURL(blob);
setSecureImageSrc(imageUrl);
// Clean up the object URL when the component unmounts
return () => URL.revokeObjectURL(imageUrl);
} catch (error) {
console.error("Error loading secure image:", error);
}
};
fetchSecureImage();
}, [src, token]);
if (!secureImageSrc) {
return <div>Loading...</div>;
}
return (
<Image
src={secureImageSrc}
alt={alt}
width={width}
height={height}
unoptimized
/>
);
};
export default SecureImage;
How It Works
- Fetch with Custom Headers: The component uses
fetch
to request the image and includes the authentication token in the headers. - Blob Conversion: The image is converted to a Blob and then to an object URL.
- Dynamic Rendering: The image is rendered using Next.js’s
Image
component with the generated object URL.
Usage Example
Here’s how you can use the SecureImage
component in your Next.js project:
function SecureContentPage() {
return (
<SecureImage
src="https://your-secure-image-url.com/image.jpg"
token="your-secure-authentication-token"
alt="Secure Content"
width={500}
height={300}
/>
);
}
Key Considerations
- Performance: This method creates a temporary object URL for each image. Use it judiciously to avoid memory leaks.
- Error Handling: Implement robust error handling for scenarios such as network failures or invalid tokens.
- Security: Always validate tokens server-side and ensure proper access controls to prevent unauthorized access.
Potential Improvements
- Add Loading Skeletons: Enhance the user experience with skeleton loaders while the image is being fetched.
- Implement Error States: Provide meaningful feedback for users when an image fails to load.
- Authentication Interceptor: Consider creating a more generic solution to handle authenticated requests across your app.
Conclusion
Secure image loading doesn’t have to be complicated. By creating a custom component like SecureImage
, you can seamlessly handle authenticated image requests while maintaining the performance benefits of Next.js’s image optimization.
Pro Tip
For even more robust security, consider implementing server-side token management to generate and validate tokens dynamically.