Why You Should Block Routes During Platform Migration in Laravel
Learn why and how to block all routes during platform migration in Laravel to ensure a smooth transition for users
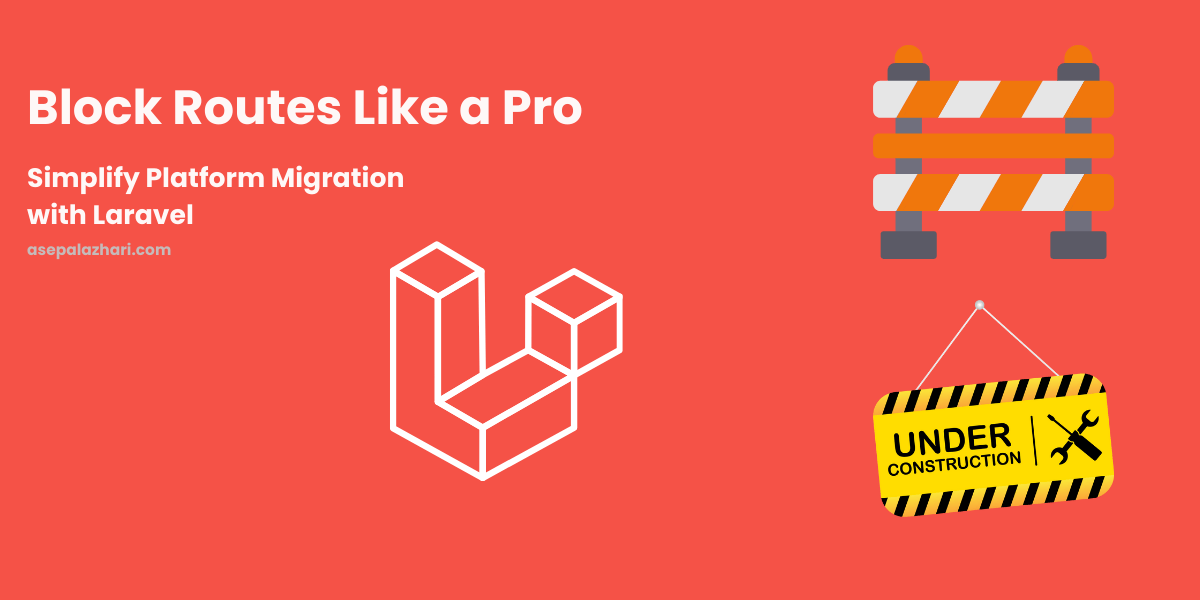
Imagine you have built a robust web app dashboard to manage data, and now it’s time to migrate to a newer, better platform. But what about users who still try to access the old system? This is where blocking routes comes in handy. Instead of leaving users confused with broken pages, you can create a single page with a clear message directing them to the new platform.
This approach ensures:
- Clear Communication: Users know where to go without frustration.
- Security: Prevents unauthorized access to outdated systems.
- Maintenance Simplification: Frees you from handling old routes and logic.
Let’s dive into how you can implement this in Laravel 8.
Implementation Steps
1. Create a Middleware
Middleware acts as a filter for HTTP requests. Create a middleware to intercept all routes.
Run this command:
php artisan make:middleware BlockRoutesMiddleware
2. Add Logic to Middleware
Modify the middleware to redirect users to a specific page unless they’re visiting the allowed route.
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class BlockRoutesMiddleware
{
public function handle(Request $request, Closure $next)
{
if (!$request->is('new-platform')) {
return redirect()->route('new-platform');
}
return $next($request);
}
}
3. Define the Notification Route
Add a route for the new platform notification page in routes/web.php
:
use Illuminate\Support\Facades\Route;
Route::get('/new-platform', function () {
return view('new-platform');
})->name('new-platform');
4. Create a Notification View
Design a simple, user-friendly Blade view for the migration notice:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>We’ve Moved!</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f4f4f4;
padding: 50px;
}
h1 {
color: #333;
}
a {
color: #007bff;
text-decoration: none;
}
</style>
</head>
<body>
<h1>We’ve Moved!</h1>
<p>Visit our new platform here:</p>
<p><a href="https://new-platform.com">Go to New Platform</a></p>
</body>
</html>
5. Register Middleware
Add the middleware to the global middleware stack in app/Http/Kernel.php
:
protected $middleware = [
// Other middleware
\App\Http\Middleware\BlockRoutesMiddleware::class,
];
6. Test It
- Verify all routes redirect to
/new-platform
except/new-platform
itself. - Ensure the notification page works perfectly.