How to Paste Plain Text in Jodit React Without Formatting Issues
Learn how to paste plain text in Jodit React without unwanted formatting. Discover best practices, configuration settings, and solutions to maintain clean and consistent content in your rich text editor
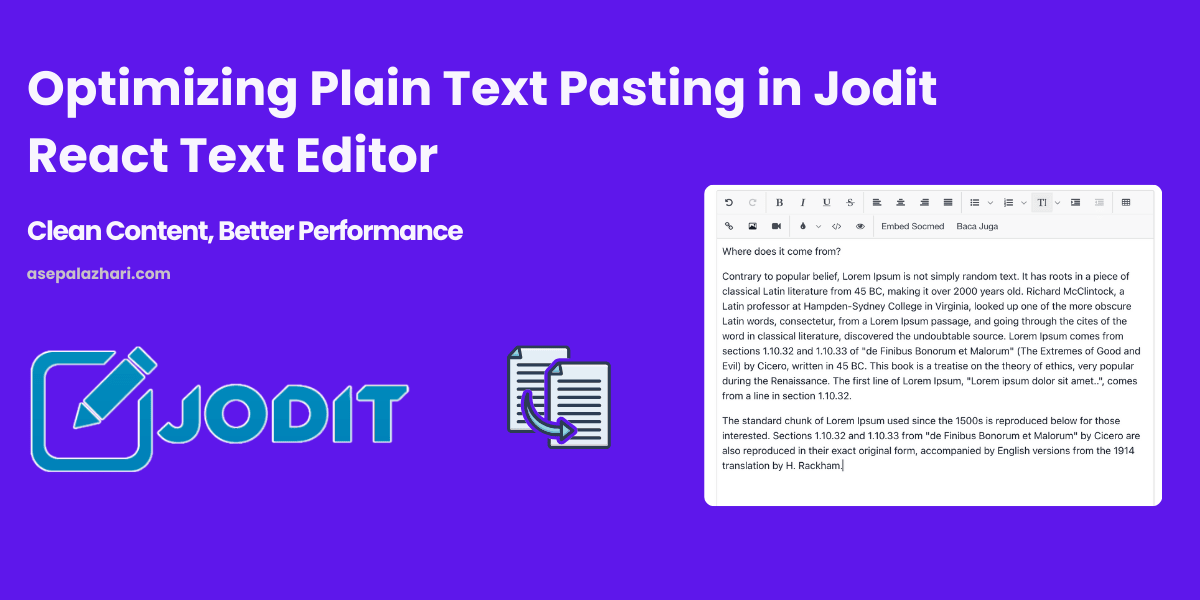
Learn how to effectively manage text formatting while pasting content in Jodit React text editor. Discover best practices, implementation techniques, and solutions to common formatting challenges for web developers and content creators.
Jodit React text editor has become an integral part of modern web applications, from content management systems to documentation tools. However, one common challenge that both developers and users face is managing text formatting when pasting content from various sources. What seems like a simple copy-paste operation can turn into a formatting nightmare, introducing unwanted styles, fonts, and HTML structures that can break your application’s design consistency.
The Copy-Paste Dilemma
Have you ever copied text from a Word document or a webpage, only to find that pasting it into your Jodit React text editor brings along a heap of unwanted formatting? This isn’t just a minor inconvenience – it can lead to serious issues such as:
- Inconsistent styling across your content
- Broken layouts due to incompatible CSS
- Increased page load times from unnecessary HTML bloat
- Accessibility issues from complex formatting structures
Understanding Paste Behaviors
Modern Jodit React text editor offers different approaches to handle pasted content. Let’s explore the main pasting mechanisms:
const editorConfig = {
// Default HTML paste
defaultActionOnPaste: "insert_as_html",
// Plain text paste
defaultActionOnPaste: "insert_only_text",
// Clean HTML paste
defaultActionOnPaste: "insert_clear_html",
};
Each of these options serves a specific purpose, and choosing the right one depends on your use case. The plain text option (insert_only_text
) strips all formatting, while clean HTML (insert_clear_html
) preserves basic structure while removing complex styling.
Also Read: Building a Universal Social Media Embed Component in React Jodit
Implementation Strategies
When implementing paste control in Jodit React text editor, consider these approaches:
- Event-Based Control: Intercept paste events to handle formatting directly.
- Configuration-Based: Use editor settings to define default behavior.
- Hybrid Approach: Combine both methods for maximum flexibility.
Here’s an example of implementing event-based paste control:
const editorConfig = {
events: {
beforePaste: (event) => {
// Prevent default paste behavior
event.preventDefault();
// Get plain text from clipboard
const text = event.clipboardData.getData("text/plain");
// Insert text at cursor position
editor.selection.insertHTML(text);
return false;
},
},
};
Best Practices for Content Creators
While technical solutions are important, establishing good content management practices is equally crucial:
- Use Keyboard Shortcuts: Familiarize yourself with shortcuts like Ctrl+Shift+V (Cmd+Shift+V on Mac) for plain text pasting.
- Content Preparation: When possible, prepare content in a plain text editor first to avoid formatting issues.
- Format After Pasting: Apply necessary formatting after pasting rather than trying to preserve original formatting.
Also Read: Best Text Editors for React: CKEditor vs TinyMCE vs Jodit
The Role of User Experience
The way your Jodit React text editor handles pasted content significantly impacts user experience. Users expect:
- Predictable behavior when pasting content
- Clear indication of what formatting will be preserved
- Easy ways to modify paste behavior when needed
- Consistent results across different content sources
Advanced Configuration Techniques
For more complex use cases, you might need to implement custom paste handling:
const customPasteHandler = {
events: {
beforePaste: (event) => {
const html = event.clipboardData.getData("text/html");
const text = event.clipboardData.getData("text/plain");
// Custom logic to clean HTML
const cleanHtml = sanitizeHtml(html, {
allowedTags: ["p", "b", "i", "a"],
allowedAttributes: {
a: ["href"],
},
});
return cleanHtml || text;
},
},
};
Looking to the Future
As web applications continue to evolve, Jodit React text editor must adapt to new challenges and user expectations. Future developments might include:
- AI-powered format cleaning
- Context-aware paste behavior
- Better handling of complex content types
- Improved cross-platform consistency
Conclusion
Managing paste behavior in Jodit React text editor is a crucial aspect of web development that impacts both user experience and content quality. By understanding the available options and implementing appropriate solutions, you can create a better editing experience for your users while maintaining clean, consistent content.
Remember that the best solution often depends on your specific use case. Consider your users’ needs, technical requirements, and maintenance capabilities when implementing paste control in Jodit React text editor.