Zustand: Lightweight State Management for Modern React Apps
Explore Zustand: The game-changing state management library for React. Learn how it simplifies complex state handling with less code and better performance.
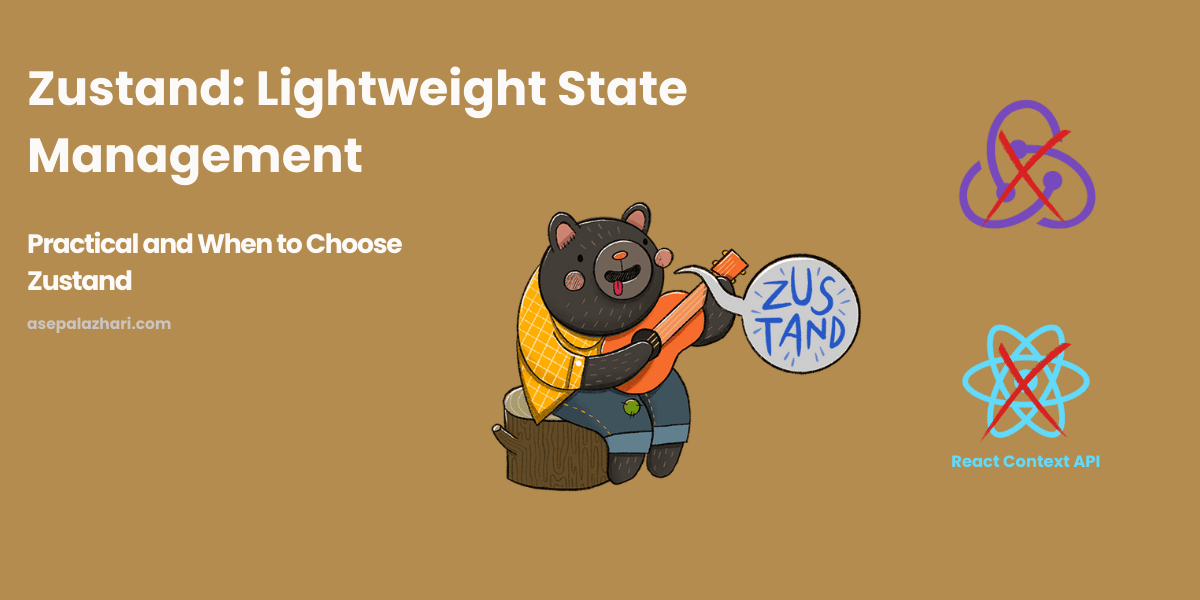
Introduction
State management is a critical aspect of building robust React applications, but choosing the right solution can be daunting. Many developers initially turn to React’s Context API, only to face performance bottlenecks as their applications grow. Others adopt Redux, praised for its predictability and power, but soon encounter its steep learning curve and boilerplate-heavy implementation.
If you’ve ever felt overwhelmed by Redux’s complexity or frustrated by the limitations of React Context, you’re not alone. Fortunately, Zustand offers a lightweight and elegant alternative that simplifies state management without sacrificing performance or flexibility.
The State Management Landscape in React
1. Traditional Approaches
React Context
- Built-in solution for prop drilling
- Simple to implement
- Performance challenges with frequent updates
- Requires multiple contexts for complex state
- Verbose wrapper components
Redux
- Robust and feature-rich
- Predictable state container
- Steep learning curve
- Boilerplate-heavy
- Requires additional libraries for async actions
- Complex setup with multiple files
Introducing Zustand: A Modern State Management Solution
Zustand stands out as a simple yet powerful library for managing state in React applications. By combining an intuitive API, minimal boilerplate, and excellent performance, it provides a refreshing alternative to traditional solutions like Redux and React Context.
Key Advantages of Zustand
1. Simplicity and Minimalism
- Lightweight library (less than 1KB)
- Intuitive API that’s easy to learn and use
- Minimal boilerplate code, enabling faster development
2. Performance Optimization
- Efficient re-rendering ensures optimal performance
- Automatic component update optimization reduces unnecessary renders
- Smaller bundle size compared to Redux
3. Flexible and Unopinionated
- Compatible with functional and class components
- Supports middleware for advanced use cases
- Seamlessly integrates with React hooks
Practical Example: Zustand in Action
Basic Store Creation
import create from "zustand";
const useCounterStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
function Counter() {
const { count, increment, decrement } = useCounterStore();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
</div>
);
}
Advanced Usage: Async Actions
const useUserStore = create((set) => ({
user: null,
loading: false,
fetchUser: async (userId) => {
set({ loading: true });
try {
const response = await fetch(`/api/users/${userId}`);
const userData = await response.json();
set({ user: userData, loading: false });
} catch (error) {
set({ loading: false, error });
}
},
}));
When to Choose Zustand
- Small to medium-sized applications
- Projects requiring quick state management setup
- Teams preferring minimal configuration
- Performance-critical applications
- Developers seeking clean, readable code
Potential Limitations
- Less suitable for extremely complex state architectures
- Fewer built-in debugging tools compared to Redux
- Smaller community compared to more established libraries
Conclusions
Zustand represents a modern approach to React state management. By prioritizing simplicity, performance, and developer experience, it offers a compelling alternative to traditional solutions like Redux and React Context.