How to Save Changes in Git Without Committing to Main in VS Code
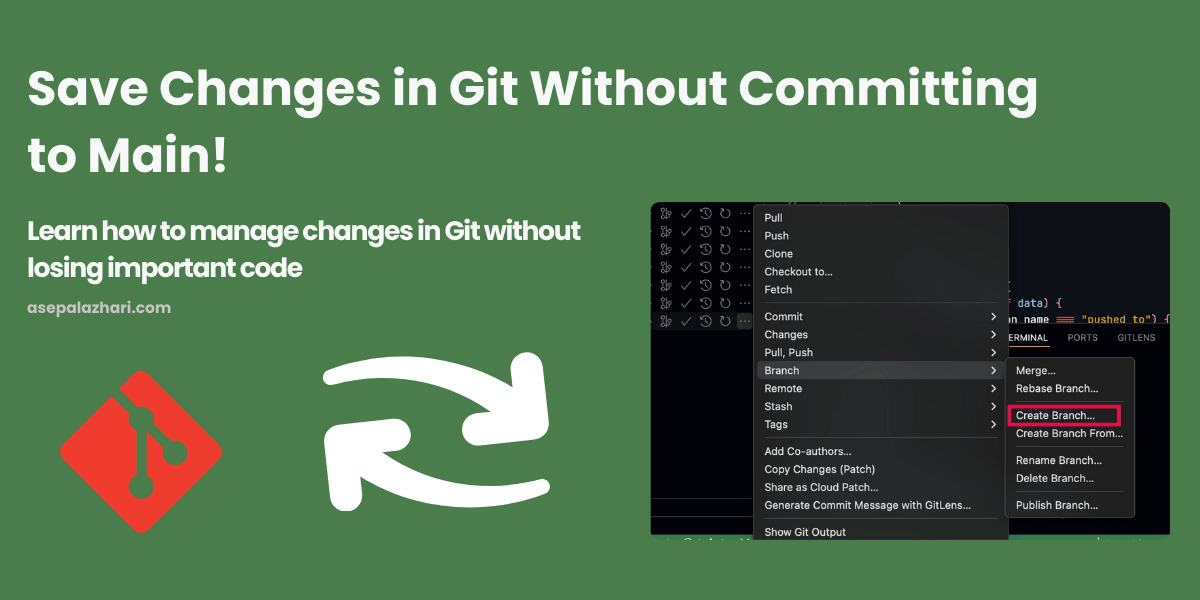
Background Story
When developing an application, sometimes we make many changes to the code but then realize that those changes are not a priority at the moment. However, we don’t want to lose them since they might be needed in the future.
For example, you are adding a new feature or fixing a bug, but suddenly an urgent task requires you to revert to the main
branch. In this situation, there are several ways to save changes without committing to main
, such as:
- Creating a new branch to store temporary changes (Recommended)
- Using
git stash
to temporarily save changes without creating a branch - Creating a patch file to save changes outside Git
This article will discuss the best way to save changes without affecting the main
branch using VS Code.
How to Save Changes Without Committing to Main
1. Creating a New Branch in VS Code (Recommended)
This method is the safest as it allows you to store changes in a separate branch and retrieve them anytime.
Steps:
- Open Visual Studio Code and make sure your Git repository is open.
- Go to the Source Control (
Ctrl + Shift + G
or click the Git icon in the left sidebar). - Click the branch name at the bottom left.
- Select "Create new branch".
- Name the branch, for example:
feature/save-changes
- Press
Enter
, and now you are in a new branch with your changes intact. - Commit the changes to this branch via Source Control or the terminal:
git add . git commit -m "Saving temporary changes"
- Switch back to
main
and discard changes if needed:git checkout main git reset --hard
If you need to restore these changes later, simply switch back to the feature/save-changes
branch:
git checkout feature/save-changes
Also Read: Efficiently Managing Git Pull Conflicts with Git Stash
2. Saving Changes with Git Stash (Alternative)
If you don’t want to create a new branch, you can use git stash
. This is a temporary method that allows you to save changes without committing.
Steps:
- Save changes to stash:
git stash push -m "Saving temporary changes"
- View the list of saved stashes:
git stash list
- Restore changes if needed:
orgit stash pop
git stash apply stash@{0}
The advantage of git stash
is that changes can be stored temporarily without creating a new branch. However, the downside is that managing multiple stashes can be tricky.
Also Read: How to Hide Git History in VS Code for a Cleaner Workspace
3. Creating a Patch File to Store Changes Outside Git
If you want to save changes without using git stash
or creating a branch, you can create a patch file.
Steps:
- Create a patch file:
git diff > saved_changes.patch
- Save the
saved_changes.patch
file in a safe location. - To reapply the changes later:
git apply saved_changes.patch
This method is useful if you want to store changes outside Git and share them with others or keep them in personal archives.
Conclusion
When code changes are not a priority at the moment, but you still want to save them for later, there are three ways to do so in Visual Studio Code:
- Creating a new branch to store changes without affecting
main
(Recommended ✅). - Using
git stash
to temporarily save changes without a branch. - Creating a patch file to store changes outside Git.
Choose the method that best suits your workflow to keep everything organized and efficient!