Solving React Query Stale Data Issues: A Step-by-Step Guide
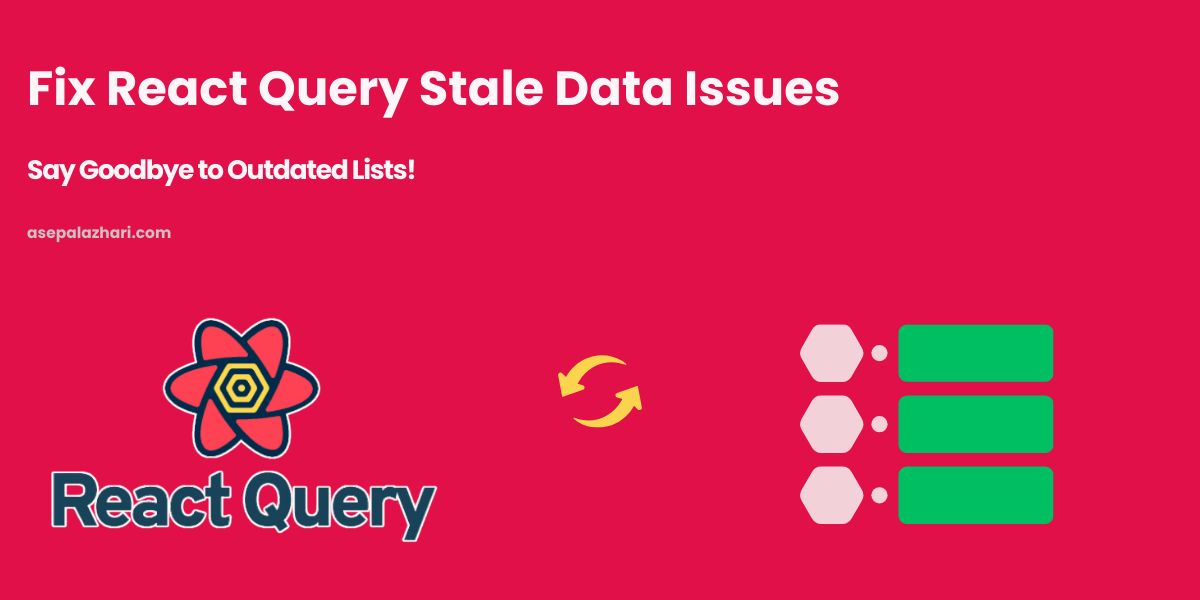
The Problem: Stale Data in React Query
Imagine this scenario: you're building a React application that fetches and displays a list of items using React Query. During testing, you notice a frustrating issue. When you first render the page, the data is either outdated or incorrect. However, if you navigate away and return to the page, the data refreshes and displays correctly. What gives?
This problem can be confusing and lead to a poor user experience. It often happens because of how React Query handles cached data and query keys. Let’s dive into the possible reasons for this issue and how to solve it step by step.
Understanding Why This Happens
React Query is a powerful library for managing server state in React. By default, it caches query results and serves cached data until new data is fetched. While this behavior improves performance, it can sometimes lead to stale or outdated data being displayed when:
- The query key isn’t unique or deterministic.
- The
staleTime
orcacheTime
settings are misconfigured. - Placeholder data is being used improperly.
- The query isn’t refetching as expected on component mount.
These behaviors are intended to optimize data fetching but require careful configuration to ensure fresh data is displayed when needed.
Also Read: Best Text Editors for React: CKEditor vs TinyMCE vs Jodit
Step-by-Step Guide to Solving Stale Data Issues
Below is the process to identify and fix stale data issues in React Query:
1. Use Unique and Deterministic Query Keys
React Query uses the query key to cache and identify data. If the query key isn’t unique or doesn’t include all dependencies, React Query might return the wrong cached data. Here's how to structure a query key properly:
const { data, isLoading } = useQuery(
["dataList", userId, page, pageSize],
fetchData
);
2. Set staleTime
Appropriately
The staleTime
setting determines how long data is considered fresh. If this value is too low, React Query might refetch unnecessarily. Conversely, if it’s too high, you’ll see stale data for longer periods.
For example, to keep data fresh for 5 minutes:
const { data, isLoading } = useQuery(
["dataList", userId, page, pageSize],
fetchData,
{
staleTime: 1000 * 60 * 5, // Data is fresh for 5 minutes
}
);
3. Avoid Placeholder Data Unless Necessary
placeholderData
provides temporary data until the query resolves. However, it doesn’t populate the cache, so it’s immediately replaced when the fetch completes. If you want to seed the cache, use initialData
instead:
const { data, isLoading } = useQuery(
["dataList", userId, page, pageSize],
fetchData,
{
initialData: {
currentPage: 0,
totalItems: 0,
data: [],
message: "",
totalPages: 0,
},
staleTime: 1000 * 60 * 5,
}
);
4. Enable Refetch on Mount or Window Focus
Sometimes, you need fresh data every time the component mounts. You can configure this behavior using refetchOnMount
or refetchOnWindowFocus
:
const { data, isLoading } = useQuery(
["dataList", userId, page, pageSize],
fetchData,
{
refetchOnMount: "always",
refetchOnWindowFocus: true,
}
);
5. Manually Invalidate the Query
If you want to ensure that stale data is never displayed, you can manually invalidate the query using React Query's useQueryClient
:
import { useQueryClient } from "@tanstack/react-query";
const queryClient = useQueryClient();
useEffect(() => {
queryClient.invalidateQueries(["dataList", userId, page, pageSize]);
}, [userId, page, pageSize]);
6. Debugging with React Query Devtools
To identify issues with your query configuration, use React Query Devtools. It provides insights into your queries, including their keys, cache state, and fetching status.
Also Read: Zustand: Lightweight State Management for Modern React Apps
Conclusion
React Query’s caching and fetching mechanisms are incredibly powerful, but they require careful handling to avoid stale data issues. By following these best practices—unique query keys, appropriate staleTime
, proper use of placeholderData
, and manual invalidation—you can ensure your application displays fresh and accurate data.
Start applying these techniques today to optimize your React Query setup and provide a seamless user experience!