Enhancing File Uploads with HEIC to JPG Conversion in React
Learn how to seamlessly handle HEIC to JPG conversion during file uploads in React applications using modern tools and techniques
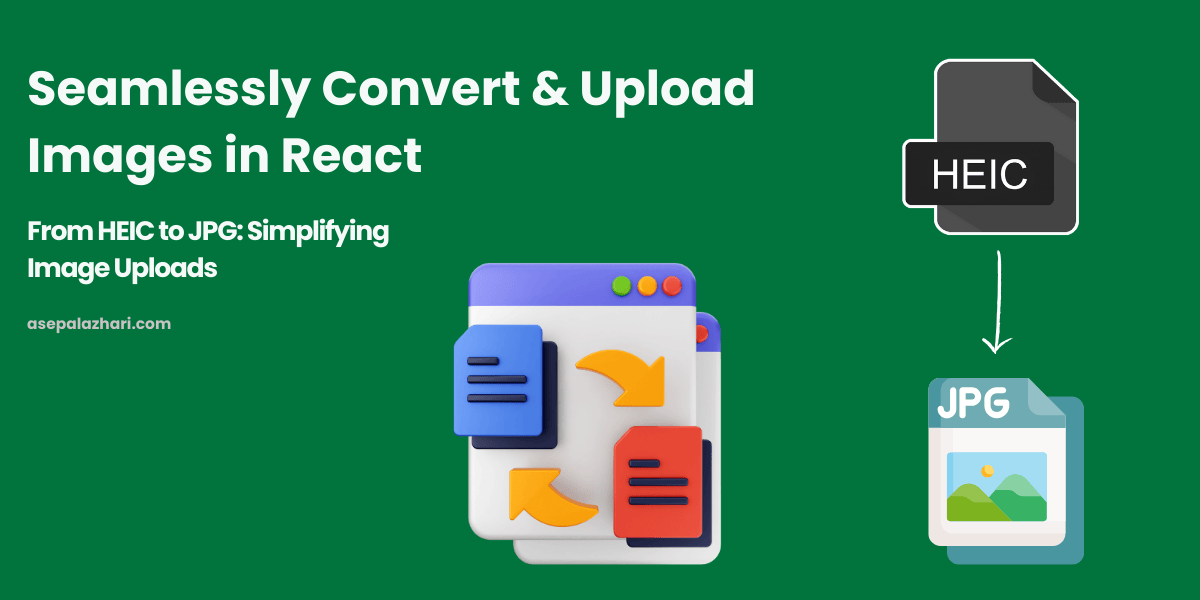
Why and When to Use HEIC to JPG Conversion in React
High Efficiency Image Format (HEIC) is popular for its superior compression and image quality compared to JPEG. However, web applications often require images in JPEG format due to better compatibility with browsers and third-party services. Integrating a seamless solution to convert HEIC files to JPG during uploads can enhance user experience and system compatibility.
Understanding the Challenge
HEIC format, widely used by Apple devices, presents a challenge for web developers. Many modern browsers do not natively support HEIC, necessitating conversion to the more universally accepted JPG format.
Also Read: Building a Universal Social Media Embed Component in React Jodit
Implementation: Setting Up a React Component for File Upload and Conversion
Below is a step-by-step guide to implement file uploads in a React application, including automatic conversion of HEIC files to JPG.
import React, { useState } from "react";
import {
Modal,
ModalOverlay,
ModalContent,
ModalHeader,
ModalFooter,
ModalBody,
ModalCloseButton,
Button,
useToast,
Input,
Progress,
Alert,
AlertIcon,
} from "@chakra-ui/react";
import { useDropzone } from "react-dropzone";
import heic2any from "heic2any";
const MAX_FILE_SIZE = 2 * 1024 * 1024;
export default function UploadMedia({ isOpen, onClose }) {
const [files, setFiles] = useState([]);
const [uploadProgress, setUploadProgress] = useState(0);
const [isLoading, setIsLoading] = useState(false);
const toast = useToast();
const handleDrop = async (acceptedFiles) => {
const processedFiles = [];
for (const file of acceptedFiles) {
if (file.size > MAX_FILE_SIZE) {
toast({
title: "File Too Large",
description: `File ${file.name} exceeds 2MB limit`,
status: "error",
duration: 7000,
position: "top",
isClosable: true,
});
continue;
}
if (file.type === "image/heic") {
try {
setIsLoading(true);
const convertedBlob = await heic2any({
blob: file,
toType: "image/jpeg",
});
const convertedFile = new File([convertedBlob], file.name.replace(/\.heic$/i, ".jpg"), {
type: "image/jpeg",
});
processedFiles.push(convertedFile);
} catch (error) {
toast({
title: "Conversion Error",
description: `Failed to convert ${file.name}`,
status: "error",
duration: 7000,
position: "top",
isClosable: true,
});
} finally {
setIsLoading(false);
}
} else {
processedFiles.push(file);
}
}
setFiles((prevFiles) => [...prevFiles, ...processedFiles]);
};
const { getRootProps, getInputProps } = useDropzone({
onDrop: handleDrop,
accept: {
"image/*": [".jpeg", ".png", ".jpg", ".gif", ".webp", ".heic"],
},
maxFiles: 10,
});
return (
<Modal isOpen={isOpen} onClose={onClose} size="lg">
<ModalOverlay />
<ModalContent>
<ModalHeader>Upload Media</ModalHeader>
<ModalCloseButton />
<ModalBody>
<div {...getRootProps()} className="dropzone">
<input {...getInputProps()} />
<p>Drag & drop files here, or click to select files.</p>
</div>
{isLoading && <Progress value={uploadProgress} size="sm" colorScheme="blue" mb={4} />}
{files.map((file, index) => (
<Alert status="info" key={index} mt={2}>
<AlertIcon />
{file.name} - {file.type === "image/jpeg" ? "Converted to JPG" : "Uploaded as is"}
</Alert>
))}
</ModalBody>
<ModalFooter>
<Button colorScheme="blue" mr={3} onClick={onClose} disabled={isLoading}>
Close
</Button>
</ModalFooter>
</ModalContent>
</Modal>
);
}
Best Practices for Image Conversion in React
- User Experience: Provide clear alerts or messages to inform users about the conversion process.
- Efficiency: Limit file size to improve upload times.
- Compatibility: Convert images to formats widely supported by browsers.
Conclusion
Efficiently managing image uploads and conversions in React applications enhances the user experience and ensures compatibility with diverse platforms. Implementing automatic conversion from HEIC to JPEG simplifies handling modern image formats while maintaining quality and reducing compatibility issues. By leveraging tools like heic2any and Chakra UI for intuitive design elements, developers can deliver robust, user-friendly solutions.
Also Read: Migrate from Bootstrap to Tailwind CSS: My Journey
For those seeking to optimize file management further, integrating additional functionalities such as drag-and-drop interfaces, file previews, and custom naming capabilities provides a polished and professional touch. The right approach to media management can significantly improve your application’s usability and performance.